In android, ScrollView is a kind of layout that is useful to add vertical or horizontal scroll bars to the content which is larger than the actual size of layouts such as linearlayout, relativelayout, framelayout, etc.
Generally, the android ScrollView is useful when we have content that doesn’t fit our android app layout screen. The ScrollView will enable a scroll to the content which is exceeding the screen layout and allow users to see the complete content by scrolling.
The android ScrollView can hold only one direct child. In case, if we want to add multiple views within the scroll view, then we need to include them in another standard layout like linearlayout, relativelayout, framelayout, etc.
To enable scrolling for our android applications, ScrollView is the best option but we should not use ScrollView along with ListView or Gridview because they both will take care of their own vertical scrolling.
In android, ScrollView supports only vertical scrolling. In case, if we want to implement horizontal scrolling, then we need to use a HorizontalScrollView component.
The android ScrollView is having a property called android:fillViewport, which is used to define whether the ScrollView should stretch it’s content to fill the viewport or not.
Now we will see how to use ScrollView with linearlayout to enable scroll view to the content which is larger than screen layout in android application with examples.
Android ScrollView Example
Following is the example of enabling vertical scrolling to the content which is larger than the layout screen using an android ScrollView object.
Create a new android application using android studio and give names as ScrollViewExample. In case if you are not aware of creating an app in android studio check this article Android Hello World App.
Once we create an application, open activity_main.xml file from \res\layout folder path and write the code like as shown below.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:fillViewport="false">
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView android:id="@+id/loginscrn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="80dp"
android:text="ScrollView"
android:textSize="25dp"
android:textStyle="bold"
android:layout_gravity="center"/>
<TextView android:id="@+id/fstTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="Welcome to Tutlane"
android:layout_gravity="center"/>
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button One" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button Two" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button Three" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button Four" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button Five" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button Six" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button Seven" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button Eight" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="60dp"
android:text="Button Nine" />
</LinearLayout>
</ScrollView>
If you observe above code, we used a ScrollView to enable the scrolling for linearlayout whenever the content exceeds layout screen.
Output of Android ScrollView Example
When we run the above example in android emulator we will get a result as shown below.

If you observe the above result, ScrollView provided a vertical scrolling for linearlayout whenever the content exceeds the layout screen.
As we discussed, ScrollView can provide only vertical scrolling for the layout. In case, if we want to enable horizontal scrolling, then we need to use HorizontalScrollView in our application.
We will see how to enable horizontal scrolling for the content which is exceeding the layout screen in the android application.
Android HorizontalScrollView Example
Now open activity_main.xml file in your android application and write the code like as shown below.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<HorizontalScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:fillViewport="true">
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal" android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginTop="150dp">
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button One" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button Two" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button Three" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button Four" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button Five" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button Six" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button Seven" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button Eight" />
<Button android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button Nine" />
</LinearLayout>
</HorizontalScrollView>
If you observe above code, we used a HorizontalScrollView to enable horizontal scrolling for linearlayout whenever the content exceeds layout screen.
Output of Android HorizontalScrollView Example
When we run the above example in the android emulator we will get a result like as shown below.
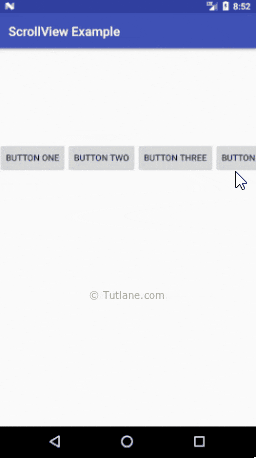
If you observe above result, HorizontalScrollView provided a horizontal scrolling for linearlayout whenever the content exceeds the layout screen.
This is how we can enable scrolling for the content which exceeds layout screen using ScrollView and HorizontalScrollView object based on our requirements.