In android, Bluetooth is a communication network protocol, which allows devices to connect wirelessly to exchange the data with other Bluetooth devices.
Generally, in android applications by using Bluetooth API’s we can implement Bluetooth functionalities, such as enable or disable a Bluetooth, searching for available Bluetooth devices, connecting with the devices and managing the data transfer between devices within the range.
In android, we can perform Bluetooth related activities by using BluetoothAdapter class in our applications. To know more about BluetoothAdapter, check this Android Bluetooth with Examples.
Android Bluetooth List Paired Devices
By using BluetoothAdapter method getBondedDevices(), we can get the Bluetooth paired devices list.
Following is the code snippet to get all paired devices with name and MAC address of each device.
// Get paired devices.
Set<BluetoothDevice> pairedDevices = bAdapter.getBondedDevices();
if (pairedDevices.size() > 0) {
// There are paired devices. Get the name and address of each paired device.
for (BluetoothDevice device : pairedDevices) {
String deviceName = device.getName();
String deviceHardwareAddress = device.getAddress(); // MAC address
}
}
If you observe above code, we are getting the Bluetooth paired devices name and mac address by using BluetoothDevice object.
As we discussed in previous tutorial Android Bluetooth with Examples, we need to set Bluetooth permissions in our android manifest file like show below to use Bluetooth features in our android applications.
<manifest ... >
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>
...
</manifest>
Following is the example of getting the list of available Bluetooth paired devices on button click in android applications.
Android List Bluetooth Paired Devices Example
Create a new android application using android studio and give names as BluetoothListPairedDevicesExample. In case if you are not aware of creating an app in android studio check this article Android Hello World App.
Once we create an application, open activity_main.xml file from \res\layout folder path and write the code like as shown below.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/btnGet"
android:text="Get Paired Devices"
android:layout_marginLeft="130dp"
android:layout_marginTop="200dp" />
<ListView
android:id="@+id/deviceList"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
</ListView>
</LinearLayout>
Now open your main activity file MainActivity.java from \java\com.tutlane.bluetoothexample path and write the code like as shown below
MainActivity.java
package com.tutlane.bluetoothlistpaireddevicesexample;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.ListView;
import android.widget.Toast;
import java.util.ArrayList;
import java.util.Set;
public class MainActivity extends AppCompatActivity {
private ListView lstvw;
private ArrayAdapter aAdapter;
private BluetoothAdapter bAdapter = BluetoothAdapter.getDefaultAdapter();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = (Button)findViewById(R.id.btnGet);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(bAdapter==null){
Toast.makeText(getApplicationContext(),"Bluetooth Not Supported",Toast.LENGTH_SHORT).show();
}
else{
Set<BluetoothDevice> pairedDevices = bAdapter.getBondedDevices();
ArrayList list = new ArrayList();
if(pairedDevices.size()>0){
for(BluetoothDevice device: pairedDevices){
String devicename = device.getName();
String macAddress = device.getAddress();
list.add("Name: "+devicename+"MAC Address: "+macAddress);
}
lstvw = (ListView) findViewById(R.id.deviceList);
aAdapter = new ArrayAdapter(getApplicationContext(), android.R.layout.simple_list_item_1, list);
lstvw.setAdapter(aAdapter);
}
}
}
});
}
}
If you observe above code, we are getting the Bluetooth paired devices name and mac address by using BluetoothDevice object.
As discussed, we need to set Bluetooth permissions in android manifest file (AndroidManifest.xml) to access Bluetooth features in android applications. Now open android manifest file (AndroidManifest.xml) and write the code like as shown below
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.tutlane.bluetoothlistpaireddevicesexample">
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
If you observe above code, we added required Bluetooth permissions in manifest file to access Bluetooth features in android applications.
Output of Android Bluetooth List Paired Devices Example
When we run the above program in the android studio we will get the result as shown below.
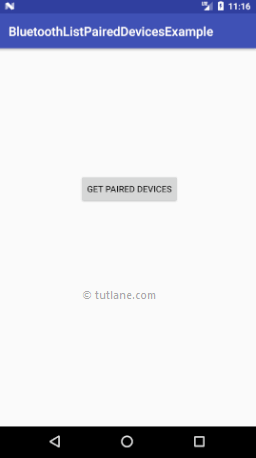
When we click on Get Paired Devices button, we will get list of paired Bluetooth devices in our android application.
This is how we can get Bluetooth paired devices list in android applications based on our requirements.