In bootstrap, modals are like popups or dialog windows to display important information on top of the current page.
The bootstrap modals are useful when we want to show the notifications or important alert messages to the users while performing actions like deleting, inserting, etc., on the current page.
Bootstrap Create a Modal
In bootstrap, we can create the modal by wrapping the modal content inside of <div>
element with .modal
class.
Following is the example of creating the modal and showing it on a button click in bootstrap.
Live Preview <!-- Button to Trigger the Modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#sampleModal">
Launch modal
</button>
<div class="modal" id="sampleModal">
<div class="modal-dialog">
<div class="modal-content">
<!--Modal Header-->
<div class="modal-header">
<h5 class="modal-title">Modal Title</h5>
<button type="button" class="close" data-dismiss="modal">
<span>×</span>
</button>
</div>
<!--Modal Body -->
<div class="modal-body">
<p>Modal body content will be here.</p>
</div>
<!--Modal Footer-->
<div class="modal-footer">
<button type="button" class="btn btn-success">Save</button>
<button type="button" class="btn btn-danger" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
If you observe the above code, we added data-target="#sampleModal"
attribute to the button to open the modal on button click.
The above example will return the result as shown below.
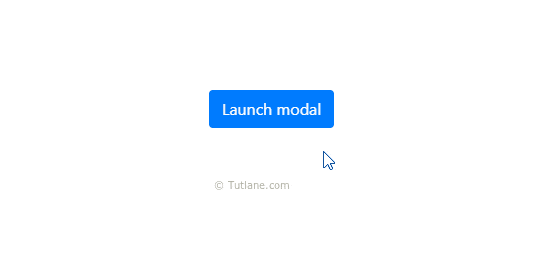
Bootstrap Animated Modal
If you observe the above example result, the modal is opening and closing without having any fade or animation effect on the button click. In case, if you want to animate or fade the modal while opening or closing, then add .fade
class to the wrapping container (<div>
) along with .modal
class.
Live Preview <!-- Button to Trigger the Modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#sampleModal">
Launch modal
</button>
<div class="modal fade" id="sampleModal">
<div class="modal-dialog">
<div class="modal-content">
<!--Modal Header-->
<div class="modal-header">
<h5 class="modal-title">Modal Title</h5>
<button type="button" class="close" data-dismiss="modal">
<span>×</span>
</button>
</div>
<!--Modal Body -->
<div class="modal-body">
<p>Modal body content will be here.</p>
</div>
<!--Modal Footer-->
<div class="modal-footer">
<button type="button" class="btn btn-success">Save</button>
<button type="button" class="btn btn-danger" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
The above example will return the result as shown below.

Bootstrap Modal Sizes
In bootstrap, by default, the modals are in medium size. In case if you want to change the modal size to small, large, or extra-large, you can use .modal-sm
, .modal-lg
, and .modal-xl
classes based on our requirements.
To change the default size of the modal, you need to add either. .modal-sm
, .modal-lg
or .modal-xl
class along with the .modal-dialog class as shown below.
Live Preview <!-- Small modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#smallModal">Small modal</button>
<div class="modal fade" id="smallModal">
<div class="modal-dialog modal-sm">
<div class="modal-content">
...
</div>
</div>
</div>
<!-- Default modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#defaultModal">Default modal</button>
<div class="modal fade" id="defaultModal">
<div class="modal-dialog">
<div class="modal-content">
...
</div>
</div>
</div>
<!-- Large modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#lgModal">Large modal</button>
<div class="modal fade" id="lgModal">
<div class="modal-dialog modal-lg">
<div class="modal-content">
...
</div>
</div>
</div>
<!-- Extra large modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#xlModal">Extra large modal</button>
<div class="modal fade" id="xlModal">
<div class="modal-dialog modal-xl">
<div class="modal-content">
...
</div>
</div>
</div>
Bootstrap Vertically Centered Modal
In bootstrap, by adding .modal-dialog-centered
class to .modal-dialog
we can center the modal vertically and horizontally on the page.
Live Preview <!-- Button to Trigger the Modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#sampleModal">
Launch modal
</button>
<div class="modal" id="sampleModal">
<div class="modal-dialog modal-dialog-centered">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Tutlane Note</h5>
<button type="button" class="close" data-dismiss="modal">
<span>×</span>
</button>
</div>
<div class="modal-body">
<p>Tutlane.com is an eLearning organization providing quality online tutorials, articles, and information related to the latest programming technologies.</p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-danger" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
Bootstrap Scrollable Modal
In bootstrap, by default, the scrollbar will be added to the page to scroll the modal when the modal's content becomes too large for the user’s device.
Live Preview <!-- Button to Trigger the Modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#sampleModal">
Launch modal
</button>
<div class="modal" id="sampleModal">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Modal with Page Scroll</h5>
<button type="button" class="close" data-dismiss="modal">
<span>×</span>
</button>
</div>
<div class="modal-body">
<p>Content Here.</p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-danger" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
In case if you want to enable a scroll for the modal content to scroll inside of the modal instead of a page, then you need to add .modal-dialog-scrollable
to .modal-dialog
.
Live Preview <!-- Button to Trigger the Modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#sampleModal">
Launch modal
</button>
<div class="modal" id="sampleModal">
<div class="modal-dialog modal-dialog-scrollable">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Modal with Page Scroll</h5>
<button type="button" class="close" data-dismiss="modal">
<span>×</span>
</button>
</div>
<div class="modal-body">
<p>Content Here.</p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-danger" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
Bootstrap Show/Hide Modal via JavaScript
If you observe the above examples, we are showing the modal on button click using data-target
attribute. Instead, we can also show/hide the modal on button click using JavaScript with a bootstrap modal()
method as shown below.
Live Preview <script>
$(function() {
// Show modal on button click
$("#btnShow").click(function() {
$("#sampleModal").modal("show");
});
// Hide modal on button click
$("#btnHide").click(function() {
$("#sampleModal").modal("hide");
});
});
</script>
You can also use toggle
property to show/hide the modal on button click using JavaScript like as shown below.
<script>
$(function() {
// Toggle the modal on button click
$("#btnShow").click(function() {
$("#sampleModal").modal("toggle");
});
});
</script>
Bootstrap Modal Events
In bootstrap, the modal classes have few events for hooking into modal functionality to fire the events during opening or closing the modal.
Following are the modal events that are available in bootstrap.
Modal Event | Description |
show.bs.modal |
This event will trigger before the modal window is shown |
shown.bs.modal |
This event will trigger after the modal window is visible to the user. |
hide.bs.modal |
This event will trigger before the modal window is hidden |
hidden.bs.modal |
This event will trigger after the modal window is hidden |
The following example uses the events to show the alert message during opening and closing the modal in bootstrap.
Live Preview <script>
$(function() {
$("#sampleModal").on('show.bs.modal', function(event) {
alert('The modal window will be shown now');
});
$("#sampleModal").on('hidden.bs.modal', function(event) {
alert('The modal window is dismissed');
});
});
</script>
This is how we can use the modals in our applications to show the important information on top of the page based on our requirements.