In the previous chapters, we learned about bootstrap forms and bootstrap form inputs to design and change the default style of form input controls with different form control styles. Now, we will learn how to use bootstrap custom form elements to customize the default controls more elegantly with examples.
Bootstrap Custom Checkboxes
To create custom checkboxes, first, you need to create a container element (<div>
) with .custom-control
and .custom-checkbox
classes to wrap the checkbox input element and corresponding label element. After that add .custom-control-input
class to <input type="checkbox">
element and add .custom-control-label
class to the corresponding label element.
In case if you use labels to add text for checkboxes, then the for
attribute value of label control must match the id
of the custom checkbox.
Live Preview <form>
<div class="custom-control custom-checkbox">
<input type="checkbox" class="custom-control-input" name="customchk" id="Checkbox1">
<label for="chkenglish" class="custom-control-label">English Language</label>
</div>
<div class="custom-control custom-checkbox">
<input type="checkbox" class="custom-control-input" name="customchk" id="Checkbox2">
<label for="chktelugu" class="custom-control-label">Telugu Language</label>
</div>
</form>
If you observe the above example, the for
attribute value of label control is same as the id
of the custom checkbox. The above example will return the result as shown below.

If you observe the above result, the default style of checkboxes changed with custom bootstrap classes.
Bootstrap Custom Radio Buttons
In bootstrap, creating the custom radio buttons is same as custom checkboxes, but the only difference is we need to add .custom-radio
class to the container element (<div>
) instead of .custom-checkbox
class.
Live Preview <form>
<div class="custom-control custom-radio">
<input type="radio" name="customrdb" id="Radio1" class="custom-control-input" checked>
<label for="rdbenglish" class="custom-control-label">English Language</label>
</div>
<div class="custom-control custom-radio">
<input type="radio" name="customrdb" id="Radio2" class="custom-control-input">
<label for="rdbtelugu" class="custom-control-label">Telugu Language</label>
</div>
</form>
The above example will return the result as shown below.

Bootstrap Inline Custom Form Controls
In case if you want to arrange the custom checkboxes and radios horizontally in same row, then you need to add .custom-control-inline
class to the wrapper element (<div>
) along with other classes (custom-control
, custom-checkbox
) like as shown below.
Live Preview <form>
<!--Horizontal Checkboxes-->
<div class="custom-control custom-checkbox custom-control-inline">
<input type="checkbox" class="custom-control-input" name="customchk" id="Checkbox1">
<label for="chkenglish" class="custom-control-label">English</label>
</div>
<div class="custom-control custom-checkbox custom-control-inline">
<input type="checkbox" class="custom-control-input" name="customchk" id="Checkbox2">
<label for="chktelugu" class="custom-control-label">Telugu</label>
</div>
<hr />
<!--Horizontal Radios-->
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" name="customrdb" id="Radio1" class="custom-control-input" checked>
<label for="rdbmale" class="custom-control-label">Male</label>
</div>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" name="customrdb" id="Radio2" class="custom-control-input">
<label for="rdbfemale" class="custom-control-label">Female</label>
</div>
</form>
The above example will return the result as shown below.
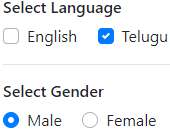
Bootstrap Disable Custom Form Controls
By adding disabled
attribute to the custom checkboxes and radio buttons, we can make the controls disabled based on our requirements.
Live Preview <form>
<div class="custom-control custom-checkbox custom-control-inline">
<input type="checkbox" class="custom-control-input" name="customchk" id="Checkbox1" disabled>
<label for="chkenglish" class="custom-control-label">English</label>
</div>
<div class="custom-control custom-checkbox custom-control-inline">
<input type="checkbox" class="custom-control-input" name="customchk" id="Checkbox2" disabled>
<label for="chktelugu" class="custom-control-label">Telugu</label>
</div>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" name="customrdb" id="Radio1" class="custom-control-input" disabled>
<label for="rdbmale" class="custom-control-label">Male</label>
</div>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" name="customrdb" id="Radio2" class="custom-control-input" disabled>
<label for="rdbfemale" class="custom-control-label">Female</label>
</div>
</form>
The above example will return the result as shown below.

Bootstrap Switches
In bootstrap, you can create the custom toggle switches same as custom checkboxes, but the only difference is you need to use .custom-switch
class instead of .custom-checkbox
in wrapper element (<div>
).
Live Preview <form>
<div class="custom-control custom-switch">
<input type="checkbox" class="custom-control-input" name="customchk" id="Checkbox1">
<label for="chkenglish" class="custom-control-label">Toggle Switch</label>
</div>
<hr />
<div class="custom-control custom-switch">
<input type="radio" name="customrdb" id="Radio1" class="custom-control-input" disabled>
<label for="rdbmale" class="custom-control-label">Disabled Switch</label>
</div>
</form>
The above example will return the result as shown below.

Bootstrap Custom Select Menu
In bootstrap, we can create the custom select menu by adding .custom-select
class to the <select>
element.
Live Preview <form>
<select class="custom-select">
<option selected>Custom Select Menu</option>
<option value="1">JavaScript</option>
<option value="2">Bootstrap</option>
<option value="3">jQuery</option>
</select>
</form>
The above example will return the result as shown below.
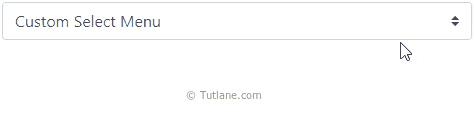
Bootstrap Custom Select Menu Sizing
In bootstrap, we can change the size of custom <select>
menu to large or small by using .custom-select-lg
, .custom-select-sm
classes.
Live Preview <!--Large Size Select Menu-->
<select class="custom-select custom-select-lg">
<option selected>Large Custom Select Menu</option>
<option value="1">JavaScript</option>
<option value="2">Bootstrap</option>
<option value="3">jQuery</option>
</select>
<!--Small Size Select Menu-->
<select class="custom-select custom-select-sm">
<option selected>Small Custom Select Menu</option>
<option value="1">JavaScript</option>
<option value="2">Bootstrap</option>
<option value="3">jQuery</option>
</select>
The above example will return the result as shown below.
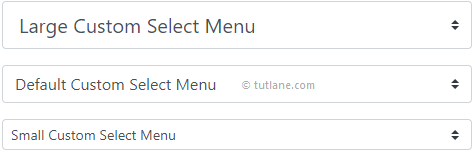
Bootstrap Custom Range Control
In bootstrap, you can create the custom range control by adding .custom-range
class to the <input type="range">
element.
Live Preview <form>
<label for="rangeinput">Set the Range</label>
<input type="range" class="custom-range" id="range1">
</form>
The above example will return the result as shown below.
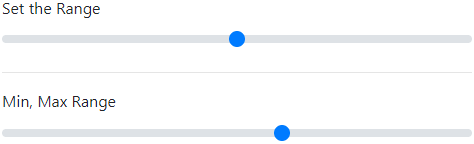
Bootstrap Custom File Upload
To create a custom file upload control, first, you need to create a container element (<div>
) with .custom-file
class to wrap the file upload element and corresponding label element. After that add .custom-file-input
class to <input type="file">
element and add .custom-file-label
class to the corresponding label element.
Live Preview <form>
<div class="custom-file">
<input type="file" class="custom-file-input" id="customFile">
<label class="custom-file-label" for="customFile">Choose file</label>
</div>
</form>
The above example will return the result as shown below.

This is how we can create the custom input controls using bootstrap custom styles based on our requirements.