The bootstrap will support all HTML input controls such as text, select, textarea, password, datetime, number, email, url, etc., and the styling available for all input controls.
By using bootstrap predefined .form-control
class, we can style textual form controls such as <input>
, <select>
and <textarea>
elements. Same way, we need to use other predefined classes such as .form-check-input
, .form-control-file
Etc. to style checkboxes, file inputs, etc., based on our requirements.
Now, we will learn how to style different input controls in bootstrap with examples.
Bootstrap Form Textual Controls
As discussed, by using .form-control
class, we can style textual form controls such as <input>
, <select>
and <textarea>
elements.
Live Preview <form>
<div class="form-group">
<label for="email">Email</label>
<input type="email" class="form-control" id="email1" placeholder="Enter email">
</div>
<div class="form-group">
<label for="password">Password</label>
<input type="password" class="form-control" id="Password1" placeholder="Enter password">
</div>
<div class="form-group">
<label for="gender">Gender</label>
<select class="form-control" id="Select1">
<option>Male</option>
<option>Female</option>
</select>
</div>
<div class="form-group">
<label for="languages">Languages</label>
<select multiple class="form-control" id="Select2">
<option>English</option>
<option>Telugu</option>
<option>Hindi</option>
<option>Kannada</option>
<option>Tamil</option>
</select>
</div>
<div class="form-group">
<label for="bio">Bio</label>
<textarea class="form-control" id="Textarea1" rows="3"></textarea>
</div>
</form>
If you observe the above example, we added .form-control
class to textual input controls to change the style of controls. The above example will return the result as shown below.
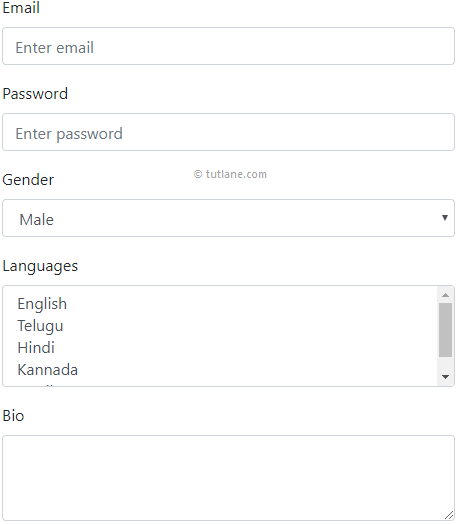
Bootstrap Form File Input Control
In bootstrap, we can style file input control by adding .form-control-file
class instead of .form-control
.
Live Preview <form>
<div class="form-group">
<label for="fileupload">Profile Pic</label>
<input type="file" class="form-control-file" id="File1">
</div>
</form>
The above example will return the result as shown below.

Bootstrap Form Checkboxes and Radios
In bootstrap, we can change the default style of checkboxes and radio controls by using .form-check
class, .form-check-input
and .form-check-label
classes.
We need to wrap all the checkboxes and radios inside of .form-check
class to provide proper spacing and alignment. The .form-check-input
class is useful to style the checkboxes and radios. The .form-check-label
class is useful to style label controls.
By default, the checkboxes and radios will be vertically stacked. Following is the example of checkboxes and radios in bootstrap.
Live Preview <form>
<div class="form-check">
<input type="radio" class="form-check-input" name="genderadios" id="Radio1" value="male" checked>
<label class="form-check-label" for="gridRadios1">Male</label>
</div>
<div class="form-check">
<input type="radio" class="form-check-input" name="genderadios" id="Radio2" value="female">
<label class="form-check-label" for="gridRadios2">Female</label>
</div>
<div class="form-check">
<input type="checkbox" class="form-check-input" name="langchk" id="Checkbox1" value="english">
<label class="form-check-label" for="english">English</label>
</div>
<div class="form-check">
<input type="checkbox" class="form-check-input" name="langchk" id="Checkbox2" value="telugu">
<label class="form-check-label" for="telugu">Telugu</label>
</div>
</form>
The above example will return the result as shown below.
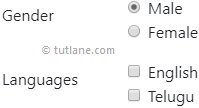
Bootstrap Inline Checkboxes and Radios
In case if you want to arrange the checkboxes and radios horizontally in the same row, then you need to add .form-check-inline
class along with .form-check
class as shown below.
Live Preview <form>
<!--Horizontal Radios-->
<div class="form-check form-check-inline">
<input type="radio" class="form-check-input" name="genderadios" id="Radio1" value="male" checked>
<label class="form-check-label" for="gridRadios1">Male</label>
</div>
<div class="form-check form-check-inline">
<input type="radio" class="form-check-input" name="genderadios" id="Radio2" value="female">
<label class="form-check-label" for="gridRadios2">Female</label>
</div>
<!--Horizontal Checkboxes-->
<div class="form-check form-check-inline">
<input type="checkbox" class="form-check-input" name="langchk" id="Checkbox1" value="english">
<label class="form-check-label" for="english">English</label>
</div>
<div class="form-check form-check-inline">
<input type="checkbox" class="form-check-input" name="langchk" id="Checkbox2" value="telugu">
<label class="form-check-label" for="telugu">Telugu</label>
</div>
</form>
The above example will return the result as shown below.
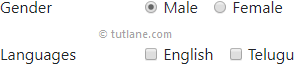
Bootstrap Form Range Input Control
In bootstrap forms, we can change the default style of range input control by adding .form-control-range
class.
Live Preview <form>
<div class="form-group">
<label for="rangeinput">Set the Range</label>
<input type="range" class="form-control-range" id="range1">
</div>
</form>
The above example will return the result as shown below.

Bootstrap Form Control Sizing
In bootstrap, we can change the size of <input>
and <select>
controls to large or small by using .form-control-lg
, .form-control-sm
classes. Same way, we can also change the size of <label>
or <legend>
controls by using .col-form-label-lg
or .col-form-label-sm
classes.
Live Preview <form>
<div class="form-group row">
<label for="email" class="col-sm-2 col-form-label col-form-label-lg">Name</label>
<div class="col-sm-10">
<input type="text" class="form-control form-control-lg" id="Text2" placeholder="Large">
</div>
</div>
<div class="form-group row">
<label for="password" class="col-sm-2 col-form-label">Location</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="Text3" placeholder="Default">
</div>
</div>
<div class="form-group row">
<label for="password" class="col-sm-2 col-form-label col-form-label-sm">Education</label>
<div class="col-sm-10">
<input type="text" class="form-control form-control-sm" id="Text4" placeholder="Small">
</div>
</div>
</form>
The above example will return the result as shown below.
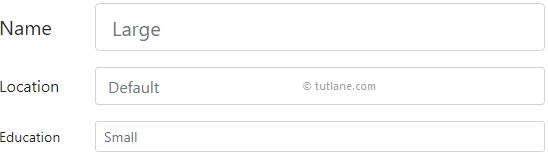
Same way, you can apply .form-control-lg
, .form-control-sm
classes on <select>
elements to change the size of controls as shown below.
<select class="form-control form-control-lg">
<option>Large</option>
</select>
<select class="form-control">
<option>Default</option>
</select>
<select class="form-control form-control-sm">
<option>Small</option>
</select>
Bootstrap Form Control States
In bootstrap, by adding disabled
and readonly
attributes to form input controls we can prevent the modification of input values.
The disabled
attribute will completely disable the input controls, and it won’t allow making any modifications to the content. The readonly
attribute is also the same as disabled
attribute, but the only difference is it will retain the standard cursor.
Live Preview <form>
<div class="form-group">
<label for="username">Username (touch to focus)</label>
<input type="text" class="form-control" id="Text1" placeholder="Enter username">
</div>
<div class="form-group">
<label for="usernameDisabled">Username (disabled)</label>
<input type="text" class="form-control" id="Text2" placeholder="Enter username" disabled>
</div>
<div class="form-group">
<label for="usernameReadonly">Username (readonly)</label>
<input type="text" class="form-control" id="Text3" placeholder="Enter username" readonly>
</div>
</form>
The above example will return the result as shown below.
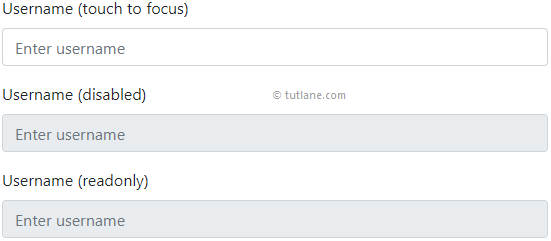
Bootstrap Form Control Plain Text
In case if you want to style the form input controls as plain text, then you need to apply .form-control-plaintext
instead of .form-control
class on <input>
controls.
The .form-control-plaintext
class will remove the default form field style and present the form input field value as plain text.
Live Preview <form>
<div class="form-group row">
<label for="email" class="col-sm-2 col-form-label">Email</label>
<div class="col-sm-10">
<input type="email" class="form-control-plaintext" id="email1" value="support@tutlane.com">
</div>
</div>
<div class="form-group row">
<label for="password" class="col-sm-2 col-form-label">Password</label>
<div class="col-sm-10">
<input type="password" class="form-control" id="Password1" placeholder="Enter password">
</div>
</div>
<div class="form-group row">
<div class="col-sm-10 offset-sm-2">
<button type="submit" class="btn btn-primary">Sign In</button>
</div>
</div>
</form>
The above example will return the result as shown below.
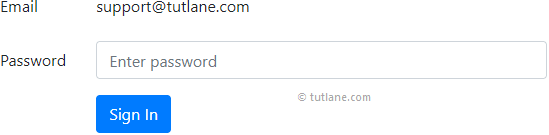
This is how we can change the default style of input controls using bootstrap built-in classes based on our requirements.