In c#, Indexer is a special type of property, and that allows instances of a class or structure to be indexed same like an array.
If we define an indexer for a class, then that class will behave like a virtual array, and we can access that class instance values without specifying a type or instance member using an array access operator ([]).
In c#, the indexer is same as the property, but the only difference is, the indexer will define this keyword along with the square bracket and parameters.
C# Indexer Syntax
Following is the syntax of defining an indexer on a class or structure using this keyword in c#.
[access_modifier] [return_type] this[parameter_type parameter_name]
{
get
{
// return specified index value
}
set
{
// set a value at the specified index
}
}
If you observe the above syntax, we defined an indexer same as properties with get and set accessors, but the only difference is we used this keyword along with the parameter list.
C# Indexer Example
Following is the example of defining an indexer in class to assign and retrieve values in c#.
using System;
namespace TutlaneExamples
{
class Users
{
// Declare an array
private string[] arr = new string[3];
// Define the indexer
public string this[int i]
{
get { return arr[i]; }
set { arr[i] = value; }
}
}
class Program
{
static void Main(string[] args)
{
Users ulist = new Users();
ulist[0] = "Suresh Dasari";
ulist[1] = "Rohini Alavala";
ulist[2] = "Trishika Dasari";
for (int i = 0; i < 3; i++)
{
Console.WriteLine(ulist[i]);
}
Console.ReadLine();
}
}
}
If you observe the above example, we implemented an indexer in the Users class to access the internal collection of a string array. We are using the Users class object ulist as an array to add or retrieve data.
When we execute the above program, we will get the result as shown below.
Suresh Dasari
Rohini Alavala
Trishika Dasari
This is how we can use indexers in our applications to allow instances of a class or structure to be indexed same as an array.
C# Overload Indexer
In c#, we can overload an indexer by creating other indexers with different index types. Following is the example of overloading an indexer with different index types.
using System;
namespace TutlaneExamples
{
class Users
{
// Declare an array
private string[] arr = new string[3];
// Define the indexer
public string this[int i]
{
get { return arr[i]; }
set { arr[i] = value; }
}
// Overload an indexer with string type
public string this[string name]
{
get
{
foreach (string str in arr)
{
if (str.ToLower() == name.ToLower())
{
return str.ToUpper();
}
}
return null;
}
}
}
class Program
{
static void Main(string[] args)
{
Users ulist = new Users();
ulist[0] = "Suresh Dasari";
ulist[1] = "Rohini Alavala";
ulist[2] = "Trishika Dasari";
// Accessing Indexer with int type
Console.WriteLine("*****Indexer with int Type*****");
for (int i = 0; i < 3; i++)
{
Console.WriteLine(ulist[i]);
}
// Accessing Indexer with string type
Console.WriteLine("*****Indexer with string Type*****");
Console.WriteLine(ulist["Suresh Dasari"]);
Console.WriteLine(ulist["Rohini Alavala"]);
Console.WriteLine(ulist["Trishika Dasari"]);
Console.ReadLine();
}
}
}
If you observe the above example, we created an indexer with string type to overload the int type indexer.
When we execute the above program, we will get the result as shown below.
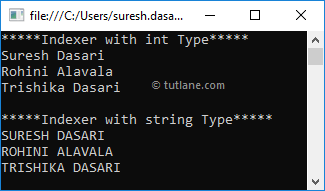
This is how we can overload an indexer with different index types based on our requirements.
C# Indexer Overview
Following are the important points which we need to remember about indexers in c#.
- In c#, the indexer is a special type of property, allowing instances of a class or structure to be indexed same as an array.
- In c#, the indexer is same as the property, but the only difference is, the indexer will define with this keyword along with the square bracket and parameters.
- Indexers can be overloaded by having different signatures.
- Indexers cannot be a static member as it’s an instance member of the class.
- Passing indexer value as a ref or out parameter is not supported.