In c#, Deserialization is the reverse process of serialization that means it will convert the stream of bytes into an object. The main purpose of deserialization is to read the stream of bytes from the file or database or memory, and we can convert it into an object.
As discussed in the previous serialization topic, we can serialize the objects either using binary or XML serialization. During the deserialization process, we need to use the same serialization pattern to convert the object into a stream of bytes.
C# Deserialization Example
Following is the example of deserializing the class which we serialized & stored in a file using binary serialization in c#.
using System;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
namespace TutlaneExamples
{
class Program
{
static void Main(string[] args)
{
UserDetails ud = new UserDetails(1, "Suresh", "Hyderabad");
Console.WriteLine("Before serialization the object contains: ");
ud.GetDetails();
string fpath = @"D:\Test.txt";
// Check if file exists
if (File.Exists(fpath))
{
File.Delete(fpath);
}
//Opens a file and serializes the object into it in binary format.
Stream stream = File.Open(fpath, FileMode.Create);
BinaryFormatter bf = new BinaryFormatter();
bf.Serialize(stream, ud);
stream.Close();
Console.WriteLine("\nSerialization Successful");
//Opens file "Test.txt" and deserializes the object from it.
stream = File.Open(fpath, FileMode.Open);
bf = new BinaryFormatter();
ud = (UserDetails)bf.Deserialize(stream);
stream.Close();
Console.WriteLine("");
Console.WriteLine("After deserialization the object contains: ");
ud.GetDetails();
Console.ReadLine();
}
}
[Serializable()]
public class UserDetails
{
public int userId { get; set; }
public string userName { get; set; }
public string location { get; set; }
public UserDetails(int id, string name, string place)
{
userId = id;
userName = name;
location = place;
}
public void GetDetails()
{
Console.WriteLine("UserId: {0}", userId);
Console.WriteLine("UserName: {0}", userName);
Console.WriteLine("Location: {0}", location);
}
}
}
If you observe the above example, we are serialized the class called “UserDetails” using binary serialization and stored it in a file. After that, we used the same binary format serialization to deserialize the object.
When we execute the above example, it will serialize the UserDetails class and stored it in the “Test.txt” file in the D drive, and return the result as shown below.
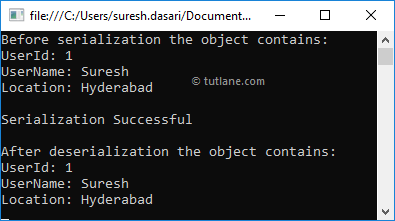
This is how we can serialize and deserialize the objects in c# based on our requirements.