In LINQ, the Cast operator is used to cast/convert all the elements present in a collection into a specified data type of new collection. In case if we try to cast/convert different types of elements (string/integer) in the collection, the conversion will fail, and it will throw an exception.
Syntax of LINQ Cast Conversion Operator
Following is the syntax of using the LINQ Cast() operator to convert items in the collection to another new collection type.
LINQ Cast Conversion Syntax in C#
IEnumerable<string> result = obj.Cast<string>();
LINQ Cast Conversion Syntax in VB.NET
Dim result As IEnumerable(Of String) = obj.Cast(Of String)()
If you observe the above syntax, we are typecasting the “result” collection to a new string object.
LINQ Cast Conversion Operator Example
Following is the example of casting or converting list/collection items into the specified data type of new collection.
C# Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Collections;
namespace LINQExamples
{
class Program
{
static void Main(string[] args)
{
ArrayList obj = new ArrayList();
obj.Add("India");
obj.Add("USA");
obj.Add("UK");
obj.Add("Australia");
IEnumerable<string> result = obj.Cast<string>();
foreach (var item in result)
{
Console.WriteLine(item);
}
Console.ReadLine();
}
}
}
VB.NET Code
Module Module1
Sub Main()
Dim obj As New ArrayList()
obj.Add("India")
obj.Add("USA")
obj.Add("UK")
obj.Add("Australia")
Dim result As IEnumerable(Of String) = obj.Cast(Of String)()
For Each item In result
Console.WriteLine(item)
Next
Console.ReadLine()
End Sub
End Module
If you observe the above example, we have an Arraylist in which we added a few countries. These countries are of type Object, and by using Cast operator, we are converting ArrayList object to string type object.
Result of LINQ Cast Operator Example
Following is the result of the LINQ Cast conversion operator example.
India
USA
UK
Australia
As discussed, the Cast operator in LINQ will convert the same type of elements present in one list to another new type. In case if the collection contains a different type of elements and if we try to convert that collection, we will get an error like “Unable to cast object of type ‘System.Int32’ to type ‘System.String’”.
C# Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Collections;
namespace LINQExamples
{
class Program
{
static void Main(string[] args)
{
ArrayList obj = new ArrayList();
obj.Add("India");
obj.Add("USA");
obj.Add("UK");
obj.Add("Australia");
obj.Add(1);
IEnumerable<string> result = obj.Cast<string>();
foreach (var item in result)
{
Console.WriteLine(item);
}
Console.ReadLine();
}
}
}
VB.NET Code
Module Module1
Sub Main()
Dim obj As New ArrayList()
obj.Add("India")
obj.Add("USA")
obj.Add("UK")
obj.Add("Australia")
obj.Add(1)
Dim result As IEnumerable(Of String) = obj.Cast(Of String)()
For Each item In result
Console.WriteLine(item)
Next
Console.ReadLine()
End Sub
End Module
If you observe the above code, we added an integer value at the end. Here we used the Cast operator to cast all the values to string, but when it reaches the integer value, it will throw an error, as shown below.
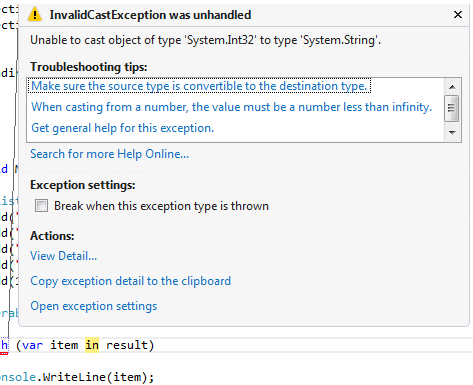