Here we will learn what is reflection in c#, uses of reflection in c#, and how to use reflection in c# with real time examples.
What is Reflection in C#?
In c#, reflection is a feature that is useful to get and modify the metadata information (assemblies, members, fields, properties, methods) of types such as classes, structs, interfaces, etc. at runtime.
By using reflection, you can create type instances dynamically at runtime, invoke its methods, access its fields and properties, and other attributes that are defined in your code.
In c#, to fetch and modify the metadata information of types, you need to use the System.Reflection
namespace.
C# Reflection Example
Following is the example of retrieving the type information of a class object using reflection in c#.
using System;
using System.Reflection;
namespace Tutlane
{
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public string Location { get; set; }
public int Salary { get; set; }
public void GetUserInfo()
{
Console.WriteLine("Get UserInfo Details");
}
public void DeleteUser()
{
Console.WriteLine("Delete User Details");
}
}
class Program
{
static void Main(string[] args)
{
User user = new User();
GetTypeDetails(user);
Console.ReadLine();
}
private static void GetTypeDetails(User user)
{
Type type = user.GetType();
Console.WriteLine("\n======Get Class Metadata Info======");
Console.WriteLine("Type Name: " + type.Name);
Console.WriteLine("Assembly Info: " + type.AssemblyQualifiedName);
Console.WriteLine("Namespace: " + type.Namespace);
Console.WriteLine("Is it Class? " + type.IsClass);
// Get Properties
Console.WriteLine("\n======Get Class Properties======");
foreach (PropertyInfo property in type.GetProperties())
{
Console.WriteLine("Property Name:" + property.Name + ", Property Type:" + property.PropertyType.Name);
}
// Get Methods
Console.WriteLine("\n======Get Class Methods======");
foreach (MethodInfo method in type.GetMethods())
{
Console.WriteLine("Method Name:" + method.Name + ", Return Type:" + method.ReturnType.Name);
}
}
}
}
In this reflection example, we are able to get the type information of the class. We used the GetProperties() method to get all the properties of the class, and GetMethods() to get all the methods of a class.
If you want to fetch fields/variables of the class, you need to use GetFields() method. In case you wish to return all members of the class use the GetMembers() method.
When you execute this reflection example, you will get the result below.
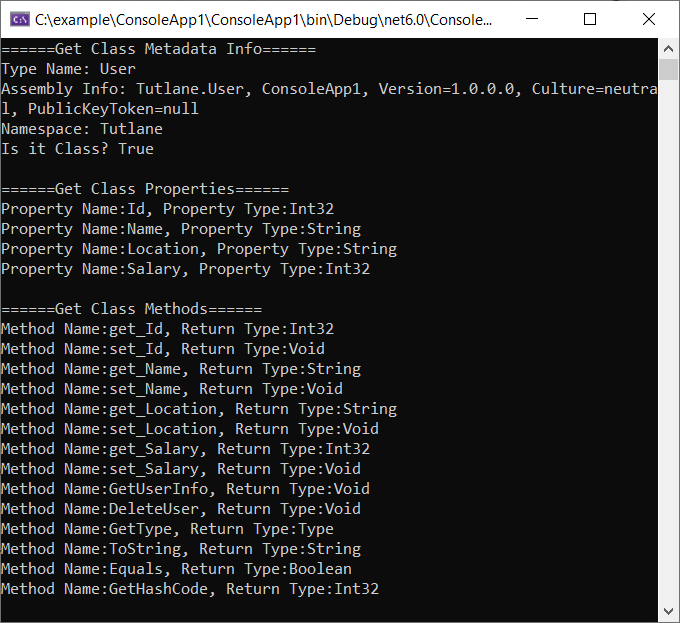
Reflection to Create Instance Dynamically in C#
By using reflection, you can create the class instance dynamically. The following is an example of creating the instance of a class dynamically using reflection in c#.
using System;
using System.Reflection;
namespace Tutlane
{
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public string Location { get; set; }
public int Salary { get; set; }
public void GetUserInfo()
{
Console.WriteLine("Get UserInfo Details");
}
public void DeleteUser()
{
Console.WriteLine("Delete User Details");
}
}
class Program
{
static void Main(string[] args)
{
Type type = typeof(User);
//Creating instance of type dynamically
object instance = Activator.CreateInstance(type);
User user = (User)instance;
user.GetUserInfo();
user.DeleteUser();
Console.ReadLine();
}
}
}
In this reflection example, we use the CreateInstance method to create the instance of a class dynamically.
When you execute this example, you will get the result below.
Get UserInfo Details
Delete User Details
Reflection to Invoke Methods Dynamically in C#
In c#, by using reflection you can invoke methods dynamically. The following is an example of invoking methods dynamically using reflection in c#.
using System;
using System.Reflection;
namespace Tutlane
{
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public string Location { get; set; }
public int Salary { get; set; }
public void GetUserInfo()
{
Console.WriteLine("Get UserInfo Details");
}
public void DeleteUser()
{
Console.WriteLine("Delete User Details");
}
}
class Program
{
static void Main(string[] args)
{
Type type = typeof(User);
object instance = Activator.CreateInstance(type);
// Invoking get method dynamically
MethodInfo getMethod = type.GetMethod("GetUserInfo");
getMethod.Invoke(instance, null);
// Invoking delete method dynamically
MethodInfo deleteMethod = type.GetMethod("DeleteUser");
deleteMethod.Invoke(instance, null);
Console.ReadLine();
}
}
}
In this reflection example, we used GetMethod and Invoke methods to get the method details and invoke the methods dynamically.
When you execute this example, you will get the result below.
Get UserInfo Details
Delete User Details
This is how we can use reflection to get the type information of properties, methods, fields, etc. of an object, create instances of an object dynamically, and invoke methods dynamically based on the requirements.
By using reflection, you can perform a wide range of operations by manipulating the type of objects, but you need to remember that the reflection can have performance problems.